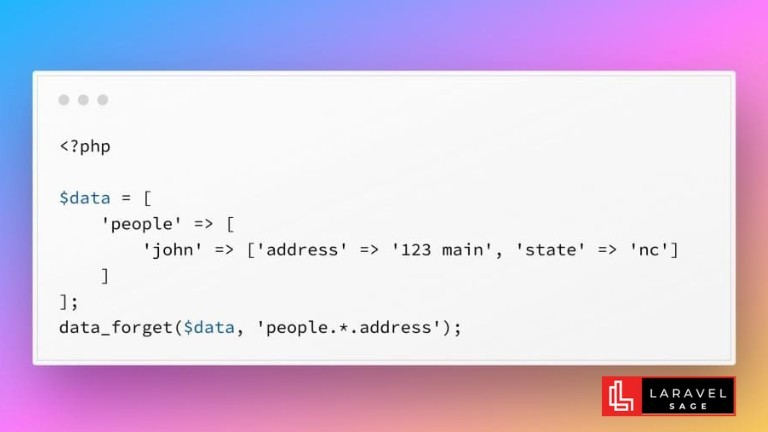
data_forget Helper for Laravel
Since Laravel version 10.15, there is a new utility function called data_forget that allows you to remove keys from an array or object using a "dot" notation.
Both React and Laravel are powerhouses in their own right. React has an excellent front-end library for creating single-page applications, while Laravel is an excellent PHP framework that is perfect for developing server-side logic. Combining these two can result in highly efficient web applications. If this is your first time deploying React with Laravel backend, it might seem tricky. Here's a guide to help you.
Before diving in, make sure you have the following installed:
PHP >= 7.3
Composer
Node.js and npm
Laravel CLI
Git
Begin by creating a new Laravel project:
composer create-project laravel/laravel .
Laravel provides an easy way to scaffold a React setup using its frontend preset:
composer require laravel/ui
php artisan ui react
Now, install the dependencies:
npm install --save-dev react react-dom
To make this usable throughout your entire application, you'll have to include this in your JavaScript code bundle. To do so, open the resoureces/js/app.js file and update its code as follows:
// resoureces/js/app.js
require('./bootstrap');
// React Components
require('./components/Example')
You'll have to change the Laravel Mix configuration so that it can compile the React component properly. To do so, open the webpack.mix.js file from the project root and update its code as follows:
// webpack.mix.js
const mix = require('laravel-mix');
mix.js('resources/js/app.js', 'public/js')
.react()
.postCss('resources/css/app.css', 'public/css', [
//
]);
You'll find a resources/js
directory where you can build your React application. The entry point is resources/js/app.js
.
Here's an example of a simple React component (resources/js/components/Example.js
):
import React from 'react';
const Example = () => {
return (
<div className="container">
<h1>Hello, React!</h1>
</div>
);
}
export default Example;
Compile your React and Laravel assets using Laravel Mix:
npm run dev
To make Laravel work with React Router, you need to ensure that all routes point to your Laravel entry point (public/index.php
). In your routes/web.php
file, add:
Route::get('/{any}', function () {
return view('welcome');
})->where('any', '.*');
Once you're satisfied with your local setup, it's time to deploy. You can use various methods for deploying a Laravel app, such as:
Shared Hosting: Zip your project and upload it via cPanel, then link your database.
AWS EC2: Provision an EC2 instance and deploy your application using Git and Composer.
Heroku: Use the Heroku CLI to deploy both the React frontend and Laravel backend easily.
Docker: Containerize your application for a consistent environment.
Remember:
Run composer install
for installing PHP dependencies.
Run npm install
and npm run prod
for your frontend dependencies and build.
Adjust your .env
settings according to your production environment.
Integrating a React frontend with a Laravel backend is a powerful way to build modern web applications. By following this guide, you should be equipped with the basic steps required to deploy this stack successfully.
Full-stack developer with a knack for Merging creativity with technical expertise for standout solutions.
Since Laravel version 10.15, there is a new utility function called data_forget that allows you to remove keys from an array or object using a "dot" notation.
The ChatGPT Laravel API Package Generator package for Laravel generates smart API mocks in Laravel using ChatGPT prompts:
You can make your Laravel app multi-tenant using the Tenancy for Laravel Tenant package. This tenancy package lets you make any Laravel application multi-tenant without rewriting it.
Are you ready to take your Laravel skills to the next level and build extraordinary applications? Look no further! In this blog post, we will unveil a treasure trove of top packages that will revolutionize your development process.
The Livewire Tables package brings dynamic tables for models to Laravel Livewire. These are some of the features which you will get out of the box:
ImageMagick is a powerful tool for image manipulation, and integrating it with PHP can enhance your web development projects.
Subscribe for 20+ new Laravel tutorials every week
You can unsubscribe at any time. You'll also get -20% off my courses!