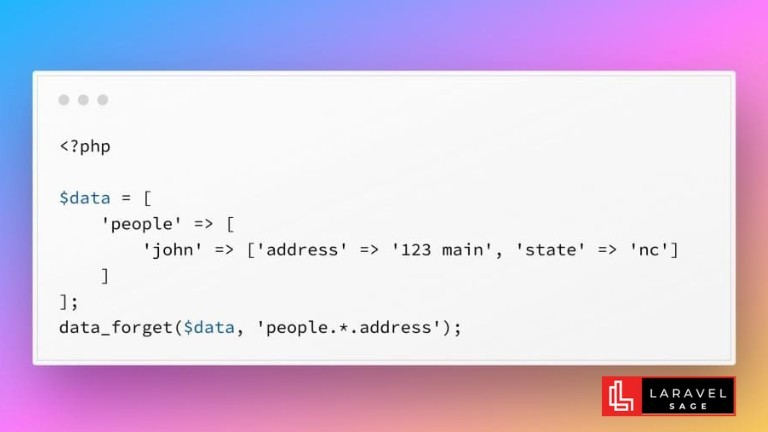
data_forget Helper for Laravel
Since Laravel version 10.15, there is a new utility function called data_forget that allows you to remove keys from an array or object using a "dot" notation.
When it comes to web development, maintaining a tidy version history of content is crucial for efficient teamwork and monitoring. With the help of Laravel and Livewire, constructing a strong versioning system is effortless. Livewire also promotes smooth communication between the frontend and backend, allowing for instantaneous updates without reloading the page. This tutorial will delve into developing an article versioning system using these tools, providing detailed instructions and necessary code snippets.
Generate models for Article
and Version
to represent articles and their versions respectively.
php artisan make:model Article -m
php artisan make:model Version -m
Establish the relationships between the Article
and Version
models.
// app/Models/Article.php
use Illuminate\Database\Eloquent\Model;
class Article extends Model
{
public function versions()
{
return $this->hasMany(Version::class);
}
}
// app/Models/Version.php
use Illuminate\Database\Eloquent\Model;
class Version extends Model
{
public function article()
{
return $this->belongsTo(Article::class);
}
}
Define and run the migrations to set up the database structure for your articles and versions.
php artisan migrate
Create Livewire components for displaying and updating articles.
php artisan make:livewire ArticleComponent
Within the ArticleComponent
, handle article updates and versioning.
/ app/Http/Livewire/ArticleComponent.php
use Livewire\Component;
use App\Models\Article;
use App\Models\Version;
class ArticleComponent extends Component
{
public $article, $content;
public function mount(Article $article)
{
$this->article = $article;
$this->content = $article->content;
}
public function update()
{
// Save current content as a new version
$version = new Version;
$version->content = $this->content;
$version->article_id = $this->article->id;
$version->save();
// Update the article's content
$this->article->content = $this->content;
$this->article->save();
}
public function render()
{
return view('livewire.article-component');
}
}
Implement a method within ArticleComponent
to handle rollback to a previous version.
// app/Http/Livewire/ArticleComponent.php
class ArticleComponent extends Component
{
// ... Other methods
public function rollback($versionId)
{
$version = Version::findOrFail($versionId);
// Save current content as a new version before rolling back
$newVersion = new Version;
$newVersion->content = $this->article->content;
$newVersion->article_id = $this->article->id;
$newVersion->save();
// Rollback to the selected version
$this->article->content = $version->content;
$this->article->save();
$this->content = $version->content;
}
}
Create a Livewire view for your ArticleComponent
to display the article, its versions, and rollback options.
<!-- resources/views/livewire/article-component.blade.php -->
<div>
<h1>{{ $article->title }}</h1>
<input type="text" wire:model="content"/>
<button wire:click="update">Update</button>
<h2>Versions</h2>
@foreach ($article->versions as $version)
<div>
<p>{{ $version->content }}</p>
<button wire:click="rollback({{ $version->id }})">Rollback to this version</button>
</div>
@endforeach
</div>
By leveraging Livewire in your Laravel project, you can build a seamless article versioning system with real-time update capabilities. This guide provides a step-by-step approach to creating such a system, effective management and tracking of content changes over time.
Full-stack developer with a knack for Merging creativity with technical expertise for standout solutions.
Since Laravel version 10.15, there is a new utility function called data_forget that allows you to remove keys from an array or object using a "dot" notation.
The ChatGPT Laravel API Package Generator package for Laravel generates smart API mocks in Laravel using ChatGPT prompts:
You can make your Laravel app multi-tenant using the Tenancy for Laravel Tenant package. This tenancy package lets you make any Laravel application multi-tenant without rewriting it.
Are you ready to take your Laravel skills to the next level and build extraordinary applications? Look no further! In this blog post, we will unveil a treasure trove of top packages that will revolutionize your development process.
The Livewire Tables package brings dynamic tables for models to Laravel Livewire. These are some of the features which you will get out of the box:
Laravel Translations UI is a package that provides a simple and friendly user interface for managing translations in a Laravel app.
Subscribe for 20+ new Laravel tutorials every week
You can unsubscribe at any time. You'll also get -20% off my courses!