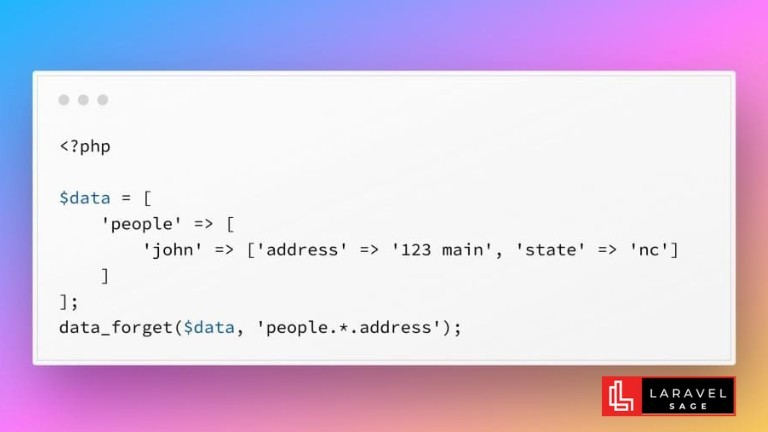
data_forget Helper for Laravel
Since Laravel version 10.15, there is a new utility function called data_forget that allows you to remove keys from an array or object using a "dot" notation.
3 min read
Tags:
In this article, we'll deep dive into implementing Laravel WebP and AVIF images solutions to optimize your application's images. Modern image formats like WebP and AVIF are no longer just 'nice-to-haves' in the era of web performance. Here is a practical approach to serving these formats in a Laravel application.
Without sacrificing quality, WebP boasts 25-35% smaller sizes than equivalent JPEGs or PNGs.
In terms of compression vs. quality, AVIF is even more efficient than WebP.
First, make sure your PHP GD library supports WebP:
composer require intervention/image
To convert an image to WebP:
use Intervention\Image\ImageManagerStatic as Image;
$image = Image::make('foo.jpg')->encode('webp', 75);
$image->save('foo.webp');
This middleware checks the Accept
header of HTTP requests to determine browser support:
namespace App\Http\Middleware;
use Closure;
class ServeWebpOrAvif
{
public function handle($request, Closure $next)
{
$acceptHeader = $request->header('Accept');
if (str_contains($acceptHeader, 'image/webp')) {
// Set a request variable to indicate WebP support
$request->merge(['preferred_image_format' => 'webp']);
} elseif (str_contains($acceptHeader, 'image/avif')) {
$request->merge(['preferred_image_format' => 'avif']);
}
return $next($request);
}
}
After creating the middleware, don't forget to register it in kernel.php
.
In your controller or route, use the request variable set by the middleware to serve the correct format:
public function serveImage(Request $request, $filename)
{
$format = $request->get('preferred_image_format', 'jpg');
$imagePath = "images/{$filename}.{$format}";
if (!file_exists($imagePath)) {
// Handle missing image, e.g., revert to JPG as default
}
return response()->file($imagePath);
}
Using the <picture>
element, you can offer multiple sources and let the browser pick the best-supported format:
<picture>
<source srcset="{{ asset('images/foo.avif') }}" type="image/avif">
<source srcset="{{ asset('images/foo.webp') }}" type="image/webp">
<img src="{{ asset('images/foo.jpg') }}" alt="Description">
</picture>
It is a forward-looking move to incorporate WebP and AVIF into Laravel, which aligns with the web's trajectory towards efficiency and speed.
The pursuit of web performance requires small tweaks, such as adopting modern image formats, to yield significant results. You can enhance your applications and broaden your web expertise by incorporating such best practices as a Laravel developer. Keep experimenting, and stay ahead of the game!🚀🖼️🌐
Full-stack developer with a knack for Merging creativity with technical expertise for standout solutions.
Since Laravel version 10.15, there is a new utility function called data_forget that allows you to remove keys from an array or object using a "dot" notation.
The ChatGPT Laravel API Package Generator package for Laravel generates smart API mocks in Laravel using ChatGPT prompts:
You can make your Laravel app multi-tenant using the Tenancy for Laravel Tenant package. This tenancy package lets you make any Laravel application multi-tenant without rewriting it.
Are you ready to take your Laravel skills to the next level and build extraordinary applications? Look no further! In this blog post, we will unveil a treasure trove of top packages that will revolutionize your development process.
The Livewire Tables package brings dynamic tables for models to Laravel Livewire. These are some of the features which you will get out of the box:
ImageMagick is a powerful tool for image manipulation, and integrating it with PHP can enhance your web development projects.
Subscribe for 20+ new Laravel tutorials every week
You can unsubscribe at any time. You'll also get -20% off my courses!